1 min to read
Playing with OCR libraries
How to recognize text from images with pytesseract library
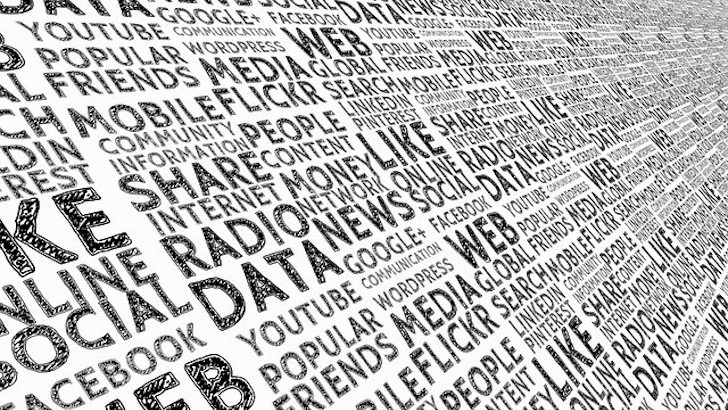
A Few days ago I found a cool library for text recognition in images, pytesseract. With this library and just a few lines of code you can implement OCR features.
Get started
Install teseract
in MacOs (for other platforms)
brew install tesseract
Create a new python environment & install requirements
python -m venv en
source env/bin/activate
pip install -r requirements.txt
requirements.txt file
numpy==1.19.4
opencv-python==4.4.0.46
Pillow==8.0.1
pytesseract==0.3.6
Create your first ocr script
from PIL import Image
import pytesseract
import argparse
import sys
original_image = "image.jpg"
image = cv2.imread(original_image)
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Convert to grayscale for better performance
filename = "grayscale_image.png"
cv2.imwrite(filename, gray)
# Load the image as a PIL/Pillow image and apply OCR
text = pytesseract.image_to_string(Image.open(filename))
os.remove(filename)
print(text)
You can find the complete code of this example in this GitHub repository